Prerequisites
- Bitcoin Core node running with RPC enabled.
- RPC credentials (username and password).
Step 1: Set Up Your HTML File
Include the axios
library in your HTML file to make HTTP requests.
<script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script>
Step 2: JavaScript Code
Below is the JavaScript code to interact with the Bitcoin Core RPC interface:
const rpcUser = 'yourusername';
const rpcPassword = 'yourpassword';
const rpcHost = 'http://127.0.0.1:8332';
async function callRPC(method, params = []) {
const response = await axios.post(rpcHost, {
jsonrpc: "1.0",
id: "curltext",
method: method,
params: params
}, {
auth: {
username: rpcUser,
password: rpcPassword
}
});
return response.data.result;
}
async function getBlockchainInfo() {
try {
const info = await callRPC('getblockchaininfo');
document.getElementById('output').innerText = JSON.stringify(info, null, 2);
} catch (error) {
console.error('Error:', error);
}
}
document.addEventListener('DOMContentLoaded', () => {
getBlockchainInfo();
});
Step 3: Display Output
The output will be displayed in the output
div below:
<div id="output"></div>
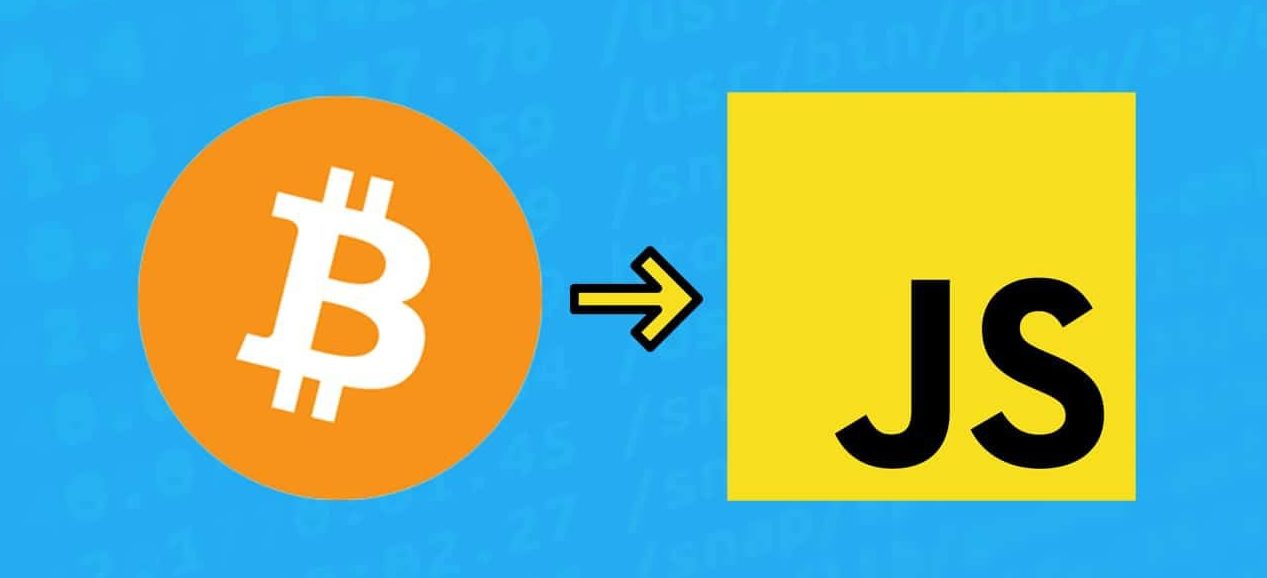